Adding a Custom Special Field in WooCommerce
Let’s add a new field to checkout, after the order notes, by hooking into the following.
/** * Add the field to the checkout */ add_action( 'woocommerce_after_order_notes', 'my_custom_checkout_field' ); function my_custom_checkout_field( $checkout ) { echo '<div id="my_custom_checkout_field"><h2>' . __('My Field') . '</h2>'; woocommerce_form_field( 'my_field_name', array( 'type' => 'text', 'class' => array('my-field-class form-row-wide'), 'label' => __('Fill in this field'), 'placeholder' => __('Enter something'), ), $checkout->get_value( 'my_field_name' )); echo '</div>'; }
Next we need to validate the field when the checkout form is posted. For this example the field is required and not optional.
/** * Process the checkout */ add_action('woocommerce_checkout_process', 'my_custom_checkout_field_process'); function my_custom_checkout_field_process() { // Check if set, if its not set add an error. if ( ! $_POST['my_field_name'] ) wc_add_notice( __( 'Please enter something into this new shiny field.' ), 'error' ); }
Finally, let’s save the new field to order custom fields using the following code.
/** * Update the order meta with field value */ add_action( 'woocommerce_checkout_update_order_meta', 'my_custom_checkout_field_update_order_meta' ); function my_custom_checkout_field_update_order_meta( $order_id ) { if ( ! empty( $_POST['my_field_name'] ) ) { update_post_meta( $order_id, 'My Field', sanitize_text_field( $_POST['my_field_name'] ) ); } }
The field is now saved to the order.
If you wish to display the custom field value on the admin order edition page, you can add this code.
/** * Display field value on the order edit page */ add_action( 'woocommerce_admin_order_data_after_billing_address', 'my_custom_checkout_field_display_admin_order_meta', 10, 1 ); function my_custom_checkout_field_display_admin_order_meta($order){ echo '<p><strong>'.__('My Field').':</strong> ' . get_post_meta( $order->id, 'My Field', true ) . '</p>'; }
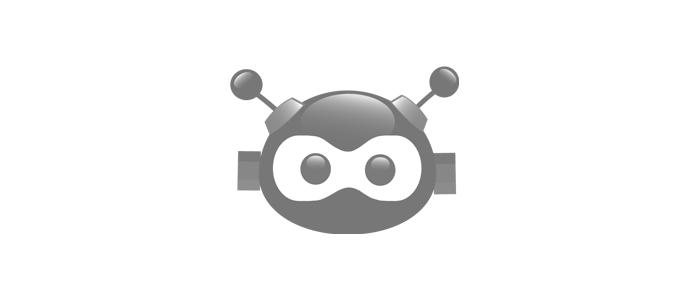